Dissolve Effect using Unity Shaders CG
- Apr 18, 2020
- 1 min read
"Mr Stark, I don't feel so good", remember this last dialogue of Peter Parker in Avengers? Remember the moment Spiderman "dissolves" in the air? Yes this is the exact effect i am talking about. Lets try to achieve this using Unity Shaders.
All you need here is to get a noise texture. What exactly is noise texture? This texture has different alpha values in it and we have to use this alpha values to create transparency gradually for our model in unity.
Lets picture this noise texture with blocks as shown below. Each block represents pixel. Each pixel has a value associated with it called "Alpha value". All you have to do is add a condition, which checks texture alpha pixel value with this noise alpha value. If it is greater than certain value(say _dissolveFactor) display that pixel, else return zero at that fragment.
So in this way by controlling the _dissolveFactor with a script(increasing/decreasing gradually) we can create this dissolve effect.
half4 frag(vertexOutput i) : COLOR { if (tex2D(_NoiseTexture,i.texcoorda).a < _dissolveFactor) return 0; else return tex2D(_Texture,i.texcoorda); }

You can download this sample noise texture here:

Ok, Here i am giving you entire code. Just copy paste it in your project for this effect:
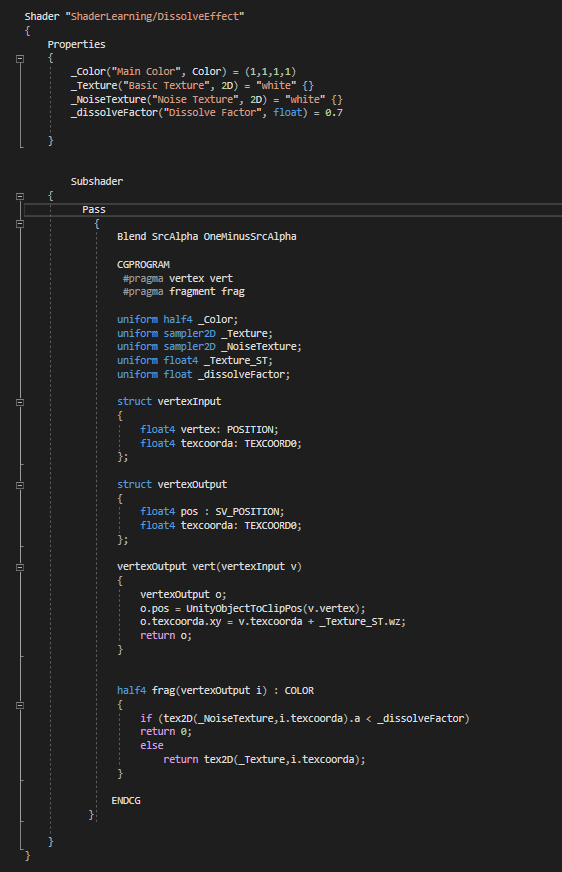
To create this fading effect over time, just include this C# code snippet.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class DissolveDemoScript : MonoBehaviour { float dissolveFactor = 0.0f; // Start is called before the first frame update void Start() { GetComponent<SkinnedMeshRenderer>().materials[0].SetFloat("_dissolveFactor", 0.0f); GetComponent<SkinnedMeshRenderer>().materials[1].SetFloat("_dissolveFactor", 0.0f); } // Update is called once per frame void Update() { if (Time.time > 2.0f) { dissolveFactor += Time.deltaTime / 5.0f; GetComponent<SkinnedMeshRenderer>().materials[0].SetFloat("_dissolveFactor", dissolveFactor); GetComponent<SkinnedMeshRenderer>().materials[1].SetFloat("_dissolveFactor", dissolveFactor); } } }
Final Effect:
Comentários